Giới thiệu sản phẩm
import pygame
import random
# Window dimensions
WIDTH = 288
HEIGHT = 512
# Bird dimensions
BIRD_WIDTH = 34
BIRD_HEIGHT = 24
# Pipe dimensions
PIPE_WIDTH = 52
PIPE_HEIGHT = 320
PIPE_GAP = 100
PIPE_VELOCITY = 2
# Initialize Pygame
pygame.init()
# Create the game window
window = pygame.display.set_mode((WIDTH, HEIGHT))
pygame.display.set_caption("Flappy Bird")
# Load game assets
bg_image = pygame.image.load("anh.png").convert()
bird_image = pygame.image.load("bird.png").convert_alpha()
pipe_image = pygame.image.load("pipe.png").convert_alpha()
# Bird class
class Bird:
def __init__(self):
self.x = WIDTH // 2 - BIRD_WIDTH // 2
self.y = HEIGHT // 2 - BIRD_HEIGHT // 2
self.vel_y = 0
self.gravity = 0.25
def jump(self):
self.vel_y = -6
def update(self):
self.vel_y += self.gravity
self.y += self.vel_y
def draw(self):
window.blit(bird_image, (self.x, self.y))
# Pipe class
class Pipe:
def __init__(self, x):
self.x = x
self.y = random.randint(HEIGHT // 2 - PIPE_HEIGHT // 2, HEIGHT // 2 + PIPE_HEIGHT // 2)
def update(self):
self.x -= PIPE_VELOCITY
def draw(self):
window.blit(pipe_image, (self.x, self.y - PIPE_HEIGHT))
window.blit(pygame.transform.flip(pipe_image, False, True), (self.x, self.y + PIPE_GAP))
# Game loop
def game_loop():
bird = Bird()
pipes = [Pipe(WIDTH + i * (WIDTH // 2)) for i in range(2)]
score = 0
clock = pygame.time.Clock()
running = True
while running:
for event in pygame.event.get():
if event.type == pygame.QUIT:
running = False
elif event.type == pygame.KEYDOWN:
if event.key == pygame.K_SPACE:
bird.jump()
bird.update()
for pipe in pipes:
pipe.update()
if pipe.x + PIPE_WIDTH < 0:
pipes.remove(pipe)
pipes.append(Pipe(WIDTH))
if bird.y > HEIGHT or bird.y < 0:
running = False
for pipe in pipes:
if bird.x + BIRD_WIDTH > pipe.x and bird.x < pipe.x + PIPE_WIDTH:
if bird.y < pipe.y or bird.y + BIRD_HEIGHT > pipe.y + PIPE_GAP:
running = False
else:
score += 1
window.blit(bg_image, (0, 0))
for pipe in pipes:
pipe.draw()
bird.draw()
pygame.display.update()
clock.tick(60)
pygame.quit()
print("Game Over! Score:", score)
# Start the game
game_loop()
Hình ảnh sản phẩm
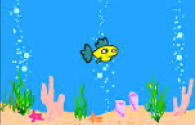
Sản phẩm cùng tác giả
Sản phẩm liên quan
Không có sản phẩm nào
bật chế độ bay lên
Đăng nhập để tham gia bình luận
Chào cả nhà
Đăng nhập để tham gia bình luận
được của ló đấy🤘🤘🤘
Đăng nhập để tham gia bình luận
👌👌 Hay quá trời quá đất
Đăng nhập để tham gia bình luận
Ảo🔥💕
Đăng nhập để tham gia bình luận
Đăng nhập để tham gia bình luận