Giới thiệu sản phẩm
from tkinter import * from tkinter import messagebox import numpy as np from turtle import * import math import random import os class login(): def __init__(self): self.parent=Tk() self.parent.geometry("500x500") self.parent.title("Trò chơi cổ điển") Label(self.parent,text="TRÒ CHƠI CỔ ĐIỂN",font=("Courier",20),bg="yellow",width=30).pack() Button(self.parent,text="Đăng kí",width=20,font=("Courier",20),height=5,bd=5,command=self.signup).pack() Label(self.parent,text="").pack() Button(self.parent,text="Đăng nhập",width=20,font=("Courier",20),height=5,bd=5,command=self.login).pack() menu=Menu(self.parent) self.parent.config(menu=menu) new_item=Menu(menu) menu.add_cascade(label="Help",menu=new_item) new_item.add_command(label="Exit",command=self.off) def login(self): global idinfo,passinfo win1=Toplevel(self.parent) win1.geometry("400x300") win1.title("ĐĂNG NHẬP") Label(win1,text="ĐĂNG NHẬP",font=("Times New Roman",20),bg="red",width=30).pack() Label(win1,text="").pack() #ID: Label(win1,text="Tên tài khoản",font=("Times New Roman",15)).place(x=10,y=90) idinfo=StringVar() id1=Entry(win1,width=35,textvariable=idinfo).place(x=140,y=90) #Password: Label(win1,text="Mật khẩu",font=("Times New Roman",15)).place(x=10,y=180) passinfo=StringVar() pass1=Entry(win1,width=35,show="*",textvariable=passinfo).place(x=140,y=180) Button(win1,text="ĐĂNG NHẬP",width=10,bg="red",bd=3,command=self.dangnhap).place(x=170,y=250) def dangnhap(self): a=idinfo.get() b=passinfo.get() listtk=os.listdir() if a in listtk: f=open(a,"r") c=f.readlines() if b in c: f.close() self.parent.destroy() run=game() else: return messagebox.showwarning("Warning","Mật khẩu sai") else: return messagebox.showerror("Error","Tên tài khoản bị sai") def signup(self): global idinfo2,passinfo2 global win2 win2=Toplevel(self.parent) win2.geometry("400x300") win2.title("ĐĂNG KÝ") Label(win2,text="ĐĂNG KÝ",font=("Times New Roman",20),bg="yellow",width=30).pack() Label(win2,text="").pack() #ID: Label(win2,text="Tên tài khoản",font=("Times New Roman",15)).place(x=10,y=90) idinfo2=StringVar() id2=Entry(win2,width=35,textvariable=idinfo2).place(x=140,y=90) #Password: Label(win2,text="Mật khẩu",font=("Times New Roman",15)).place(x=10,y=180) passinfo2=StringVar() pass2=Entry(win2,width=35,show="*",textvariable=passinfo2).place(x=140,y=180) Button(win2,text="ĐĂNG KÝ",bd=3,width=10,bg="yellow",command=self.dangky).place(x=170,y=250) def dangky(self): messagebox.showinfo("Kết quả","Đăng kí thành công") a=idinfo2.get() b=passinfo2.get() f=open(a,"w") f.write(a+" ") f.write(b) f.close() win2.destroy() def off(self): a=messagebox.askyesno("Thoát","Bạn có muốn thoát không") if a==True: self.parent.destroy() else: False class game(): def __init__(self): self.wins=Tk() self.wins.geometry("525x525") self.wins.title("Trò chơi cổ điển") Label(self.wins,text="TRÒ CHƠI CỔ ĐIỂN",font=("Courier",20),bg="yellow",width=30).pack() Button(self.wins,text="Bóng bàn",width=20,font=("Courier",15),height=5,bd=5,command=self.bongban).place(x=5,y=37) Button(self.wins,text="Tic tac toe",width=20,font=("Courier",15),height=5,bd=5,command=self.xo).place(x=265,y=37) Button(self.wins,text="CB ko gian",width=20,font=("Courier",15),height=5,bd=5,command=self.cbkg).place(x=5,y=177) Button(self.wins,text="NO",width=20,font=("Courier",15),height=5,bd=5,command=self.no).place(x=265,y=177) def bongban(self): sc=Screen() sc.title("Bóng bàn") sc.bgcolor("white") sc.setup(width=1000,height=600) vtrai=Turtle() vtrai.color("black") vtrai.shape("square") vtrai.speed(0) vtrai.shapesize(stretch_wid=6,stretch_len=2) vtrai.pu() vtrai.goto(-400,0) vphai=Turtle() vphai.color("black") vphai.shape("square") vphai.speed(0) vphai.shapesize(stretch_wid=6,stretch_len=2) vphai.pu() vphai.goto(400,0) bong=Turtle() bong.color("red") bong.shape("circle") bong.speed(1.25) bong.pu() bong.goto(0,0) bong.dx=5 bong.dy=-5 nchoi1=0 nchoi2=0 tso=Turtle() tso.ht() tso.pu() tso.speed(0) tso.color("green") tso.goto(0,260) tso.write("Người chơi 1:0 Người chơi 2:0",align="center",font=("Century",24)) def up1(): y=vtrai.ycor() y+=20 vtrai.sety(y) def down1(): y=vtrai.ycor() y-=20 vtrai.sety(y) def up2(): y=vphai.ycor() y+=20 vphai.sety(y) def down2(): y=vphai.ycor() y-=20 vphai.sety(y) sc.listen() sc.onkey(up1,"w") sc.onkey(down1,"s") sc.onkey(up2,"Up") sc.onkey(down2,"Down") while True: sc.update() bong.setx(bong.xcor() + bong.dx) bong.sety(bong.ycor() + bong.dy) if bong.ycor() > 280: bong.sety(280) bong.dy *= -1 if bong.ycor() < -280: bong.sety(-280) bong.dy *= -1 if bong.xcor() > 500: bong.goto(0, 0) bong.dy *= -1 nchoi1 += 1 tso.clear() tso.goto(0, 260) tso.write("Người chơi 1 : {} Người chơi 2: {}".format(nchoi1, nchoi2), align="center",font=("Century", 24, )) if bong.xcor() < -500: bong.goto(0, 0) bong.dy *= -1 nchoi2 += 1 tso.clear() tso.goto(0, 260) tso.write("Người chơi 1 : {} Người chơi 2: {}".format(nchoi1, nchoi2), align="center",font=("Century", 24, )) if (bong.xcor() > 360 and bong.xcor() < 370) and (bong.ycor() < vphai.ycor() + 70 and bong.ycor() > vphai.ycor() - 70): bong.setx(360) bong.dx *= -1 if (bong.xcor() < -360 and bong.xcor() > -370) and (bong.ycor() < vtrai.ycor() + 70 and bong.ycor() > vtrai.ycor() - 70): bong.setx(-360) bong.dx *= -1 def xo(self): size_of_board = 800 symbol_size = (size_of_board / 3 - size_of_board / 8) / 2 symbol_thickness = 50 symbol_X_color = '#EE4035' symbol_O_color = '#0492CF' Green_color = '#7BC043' class Tic_Tac_Toe(): def __init__(self): self.window = Tk() self.window.title('Tic-Tac-Toe') self.canvas = Canvas(self.window, width=size_of_board, height=size_of_board) self.canvas.pack() self.window.bind('<Button-1>', self.click) self.initialize_board() self.player_X_turns = True self.board_status = np.zeros(shape=(3, 3)) self.player_X_starts = True self.reset_board = False self.gameover = False self.tie = False self.X_wins = False self.O_wins = False self.X_score = 0 self.O_score = 0 self.tie_score = 0 def mainloop(self): self.window.mainloop() def initialize_board(self): for i in range(2): self.canvas.create_line((i + 1) * size_of_board / 3, 0, (i + 1) * size_of_board / 3, size_of_board) for i in range(2): self.canvas.create_line(0, (i + 1) * size_of_board / 3, size_of_board, (i + 1) * size_of_board / 3) def play_again(self): self.initialize_board() self.player_X_starts = not self.player_X_starts self.player_X_turns = self.player_X_starts self.board_status = np.zeros(shape=(3, 3)) def draw_O(self, logical_position): logical_position = np.array(logical_position) grid_position = self.convert_logical_to_grid_position(logical_position) self.canvas.create_oval(grid_position[0] - symbol_size, grid_position[1] - symbol_size, grid_position[0] + symbol_size, grid_position[1] + symbol_size, width=symbol_thickness, outline=symbol_O_color) def draw_X(self, logical_position): grid_position = self.convert_logical_to_grid_position(logical_position) self.canvas.create_line(grid_position[0] - symbol_size, grid_position[1] - symbol_size, grid_position[0] + symbol_size, grid_position[1] + symbol_size, width=symbol_thickness, fill=symbol_X_color) self.canvas.create_line(grid_position[0] - symbol_size, grid_position[1] + symbol_size, grid_position[0] + symbol_size, grid_position[1] - symbol_size, width=symbol_thickness, fill=symbol_X_color) def display_gameover(self): if self.X_wins: self.X_score += 1 text = 'Winner: Player 1 (X)' color = symbol_X_color elif self.O_wins: self.O_score += 1 text = 'Winner: Player 2 (O)' color = symbol_O_color else: self.tie_score += 1 text = 'Its a tie' color = 'gray' self.canvas.delete("all") self.canvas.create_text(size_of_board / 2, size_of_board / 3, font="cmr 60 bold", fill=color, text=text) score_text = 'Scores ' self.canvas.create_text(size_of_board / 2, 5 * size_of_board / 8, font="cmr 40 bold", fill=Green_color, text=score_text) score_text = 'Player 1 (X) : ' + str(self.X_score) + ' ' score_text += 'Player 2 (O): ' + str(self.O_score) + ' ' score_text += 'Tie : ' + str(self.tie_score) self.canvas.create_text(size_of_board / 2, 3 * size_of_board / 4, font="cmr 30 bold", fill=Green_color, text=score_text) self.reset_board = True score_text = 'Click to play again ' self.canvas.create_text(size_of_board / 2, 15 * size_of_board / 16, font="cmr 20 bold", fill="gray", text=score_text) def convert_logical_to_grid_position(self, logical_position): logical_position = np.array(logical_position, dtype=int) return (size_of_board / 3) * logical_position + size_of_board / 6 def convert_grid_to_logical_position(self, grid_position): grid_position = np.array(grid_position) return np.array(grid_position // (size_of_board / 3), dtype=int) def is_grid_occupied(self, logical_position): if self.board_status[logical_position[0]][logical_position[1]] == 0: return False else: return True def is_winner(self, player): player = -1 if player == 'X' else 1 for i in range(3): if self.board_status[i][0] == self.board_status[i][1] == self.board_status[i][2] == player: return True if self.board_status[0][i] == self.board_status[1][i] == self.board_status[2][i] == player: return True if self.board_status[0][0] == self.board_status[1][1] == self.board_status[2][2] == player: return True if self.board_status[0][2] == self.board_status[1][1] == self.board_status[2][0] == player: return True return False def is_tie(self): r, c = np.where(self.board_status == 0) tie = False if len(r) == 0: tie = True return tie def is_gameover(self): self.X_wins = self.is_winner('X') if not self.X_wins: self.O_wins = self.is_winner('O') if not self.O_wins: self.tie = self.is_tie() gameover = self.X_wins or self.O_wins or self.tie if self.X_wins: print('X wins') if self.O_wins: print('O wins') if self.tie: print('Its a tie') return gameover def click(self, event): grid_position = [event.x, event.y] logical_position = self.convert_grid_to_logical_position(grid_position) if not self.reset_board: if self.player_X_turns: if not self.is_grid_occupied(logical_position): self.draw_X(logical_position) self.board_status[logical_position[0]][logical_position[1]] = -1 self.player_X_turns = not self.player_X_turns else: if not self.is_grid_occupied(logical_position): self.draw_O(logical_position) self.board_status[logical_position[0]][logical_position[1]] = 1 self.player_X_turns = not self.player_X_turns if self.is_gameover(): self.display_gameover() else: self.canvas.delete("all") self.play_again() self.reset_board = False game_instance = Tic_Tac_Toe() game_instance.mainloop() def cbkg(self): sc=Screen() sc.setup(600,600) sc.bgcolor("black") class anhhung(Turtle): def __init__(self): Turtle.__init__(self) self.pu() self.speed(0) self.shape("arrow") self.color("red") self.speed=1 def move(self): self.fd(self.speed) if self.xcor()>280: self.setx(280) self.right(60) if self.xcor()<-280: self.setx(-280) self.right(60) if self.ycor()>280: self.sety(280) self.right(60) if self.ycor()<-280: self.sety(-280) self.right(60) def turnright(self): self.right(25) def turnleft(self): self.left(25) def tangtoc(self): self.speed+=1 def giamtoc(self): self.speed-=1 class quaivat(Turtle): def __init__(self): Turtle.__init__(self) self.pu() self.speed(0) self.shape("circle") self.color("blue") self.speed=random.randint(3,6) self.goto(random.randint(-300,300),random.randint(-300,300)) self.setheading(random.randint(0,360)) def qv_move(self): self.fd(self.speed) if self.xcor()>280: self.setx(280) self.right(60) if self.xcor()<-280: self.setx(-280) self.right(60) if self.ycor()>280: self.sety(280) self.right(60) if self.ycor()<-280: self.sety(-280) self.right(60) def qv_jump(self): self.goto(random.randint(-300,300),random.randint(-300,300)) self.setheading(random.randint(0,360)) class vien(Turtle): def __init__(self): Turtle.__init__(self) self.pu() self.speed(0) self.color("white") self.pensize(5) self.ht() def vevien(self): Turtle.__init__(self) self.pu() self.color("white") self.pensize(3) self.goto(300,300) self.pd() self.goto(-300,300) self.goto(-300,-300) self.goto(300,-300) self.goto(300,300) self.ht() def vacham(t1,t2): d=math.sqrt(math.pow(t1.xcor()-t2.xcor(),2)+math.pow(t1.ycor()-t2.ycor(),2)) if d<20: return True else: return False dv=vien() dv.vevien() player=anhhung() sc.listen() sc.onkey(player.turnright,"Right") sc.onkey(player.turnleft,"Left") sc.onkey(player.tangtoc,"Up") sc.onkey(player.giamtoc,"Down") qv=[] qv.append(quaivat()) while True: player.move() for quaivat in qv: quaivat.qv_move() if vacham(player,quaivat): quaivat.qv_jump() def no(self): a=Tk() Label(a,text="KO có gi").pack() d=login()
Đầu tiên vào sẽ đăng kí rồi đăng nhập
Sẽ có 4 phần trò chơi hiện ra
Phần No ko có gì cả
phần bóng bàn hoặc chiến bi ko gian chỉ chơi được 1 lần
nếu muốn chơi lại thì chạy lại
Sẽ có 4 phần trò chơi hiện ra
Phần No ko có gì cả
phần bóng bàn hoặc chiến bi ko gian chỉ chơi được 1 lần
nếu muốn chơi lại thì chạy lại
Hình ảnh sản phẩm
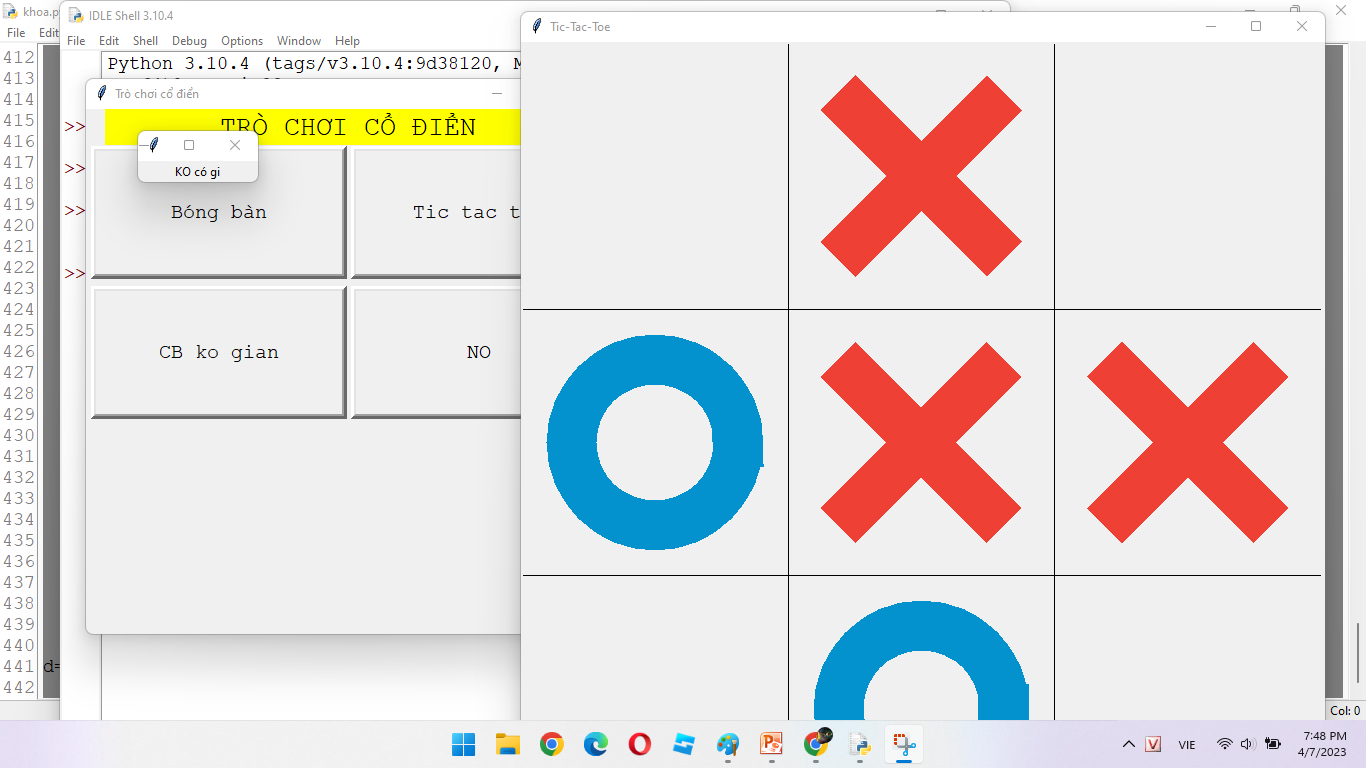
Sản phẩm cùng tác giả
Sản phẩm liên quan
Không có sản phẩm nào
Ố
Đăng nhập để tham gia bình luận
m
Đăng nhập để tham gia bình luận
tốt
Đăng nhập để tham gia bình luận
tốt
Đăng nhập để tham gia bình luận
tốt
Đăng nhập để tham gia bình luận
Đăng nhập để tham gia bình luận